This tutorial provides the steps to initialize OwlCarousel on posts output by Easy Posts element in Oxygen.
We shall
- register a custom size for the images in the carousel
- edit the PHP template to use our custom image size rather than the full-sized images and to show just the featured image and title
- add the CSS classes needed for Owl via jQuery to .oxy-posts inside our Easy Posts output
- initialize Owl such that 1 item is shown up to 499px, 2 items from 500px, 3 items from 768px and 4 items from 1024px and above.
Step 1
Install and activate my custom functionality plugin.
Upload owl.carousel.min.css and owl.theme.default.min.css to plugin’s assets/css
directory.
Upload owl.carousel.min.js to plugin’s assets/js
directory.
Inside plugin.php’s custom_enqueue_files(), add
wp_enqueue_style(
'owl-main',
plugin_dir_url( __FILE__ ) . 'assets/css/owl.carousel.min.css',
array(),
'2.3.4'
);
wp_enqueue_style(
'owl-default-theme',
plugin_dir_url( __FILE__ ) . 'assets/css/owl.theme.default.min.css',
array(),
'2.3.4'
);
wp_enqueue_script(
'owl',
plugin_dir_url( __FILE__ ) . 'assets/js/owl.carousel.min.js',
array( 'jquery' ),
'2.3.4',
true
);
You might want to place the above inside an if conditional to restrict the assets to load only on specific pages/views of your site.
At the end of the file, add
add_image_size( 'carousel-image', 600, 400, true );
Step 2
Edit your template/Page/Post in Oxygen and add a Section and inside that, Easy Posts and Code Block elements.
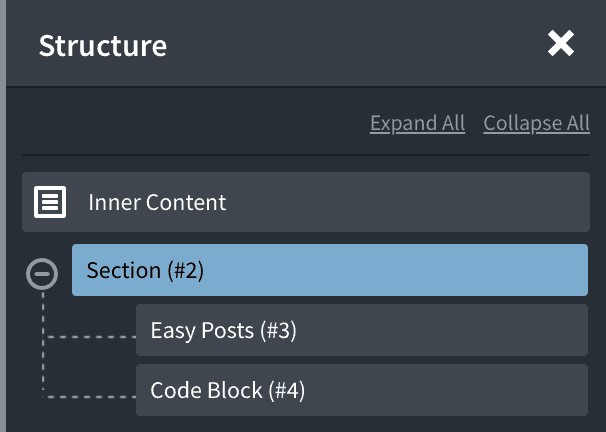
Easy Posts
Add a class of say, posts-carousel
.
Go to Query > Custom.
Set Post Type to what you want to show. In my test site, I’ve selected portfolio
.
Set the number of entries to show in the Count area. I’ve set it to 6.
Styles > Title: You might want to set the font size to say, 20px.
Template PHP:
<div class='oxy-post'>
<?php
if (has_post_thumbnail()) {
?>
<a href='<?php the_permalink(); ?>'><img src='<?php echo wp_get_attachment_image_url( get_post_thumbnail_id(), 'carousel-image' ); ?>' class='oxy-post-image' /></a>
<?php
}
?>
<a class='oxy-post-title' href='<?php the_permalink(); ?>'><?php the_title(); ?></a>
</div>
Template CSS:
%%EPID%% .oxy-post {
display: flex;
flex-direction: column;
text-align: center;
align-items: center;
margin-bottom: 2em;
}
%%EPID%% .oxy-post-image {
margin-top: 1em;
margin-bottom: 1em;
width: 100%;
}
%%EPID%% .oxy-post-title {
font-size: 2em;
line-height: 1.2em;
}
Code Block
PHP & HTML:
<?php
// echo "hello world!";
?>
CSS:
.owl-carousel {
position: relative;
}
.owl-theme .owl-nav {
margin-top: 0;
}
.owl-carousel .owl-nav button.owl-prev,
.owl-carousel .owl-nav button.owl-next {
position: absolute;
top: 70px;
padding: 10px !important;
line-height: 1;
}
.owl-carousel .owl-nav button.owl-prev:hover,
.owl-carousel .owl-nav button.owl-next:hover {
background: none;
color: #404040;
}
.owl-carousel .owl-nav .owl-prev span,
.owl-carousel .owl-nav .owl-next span {
font-size: 40px;
}
.owl-carousel .owl-nav .owl-prev {
left: -50px;
}
.owl-carousel .owl-nav .owl-next {
right: -50px;
}
Javascript:
jQuery('.posts-carousel .oxy-posts').addClass( 'owl-carousel owl-theme' );
jQuery('.owl-carousel').owlCarousel({
responsive: {
// breakpoint from 0 and up
0: {
items: 1
},
// breakpoint from 500 and up
500: {
items: 2,
margin: 20
},
// breakpoint from 768 and up
768: {
items: 3,
margin: 20
},
// breakpoint from 1024px and up
1024: {
items: 4,
margin: 20,
nav: true,
slideBy: 3,
rewind: false,
// autoplay: true,
// autoplaySpeed: 1500
}
}
});