How to override WooCommerce templates using a custom functionality plugin
My preferred method to make customizations in WooCommerce is using actions and filters. But there could be some situations where it makes sense to duplicate the WooCommerce templates to the active child theme and edit them.
What if you don’t want to or can’t (like when using Oxygen) override from within the theme and instead want to use a custom plugin though?
Here’s how it can be done.
Step 1
Install and activate My Custom Functionality plugin.
Step 2
Using a FTP client or cPanel file manager, navigate to
/wp-content/plugins/my-custom-functionality-master
Create a directory called woocommerce
.
Copy the file(s) you want to override from /wp-content/plugins/woocommerce/templates
while maintaining the folder structure.
Ex.: To override cart.php
, you would copy
/wp-content/plugins/woocommerce/templates/cart/cart.php
to
/wp-content/plugins/my-custom-functionality-master/woocommerce/cart/cart.php
while creating the directories as needed (in this example, “cart”).
Step 3
Add the following in
/wp-content/plugins/my-custom-functionality-master/plugin.php
:
add_filter( 'woocommerce_locate_template', 'intercept_wc_template', 10, 3 );
/**
* Filter the cart template path to use cart.php in this plugin instead of the one in WooCommerce.
*
* @param string $template Default template file path.
* @param string $template_name Template file slug.
* @param string $template_path Template file name.
*
* @return string The new Template file path.
*/
function intercept_wc_template( $template, $template_name, $template_path ) {
if ( 'cart.php' === basename( $template ) ) {
$template = trailingslashit( plugin_dir_path( __FILE__ ) ) . 'woocommerce/cart/cart.php';
}
return $template;
}
Replace cart.php
and cart/cart.php
in woocommerce/cart/cart.php
as needed.
To override multiple files:
add_filter( 'woocommerce_locate_template', 'intercept_wc_template', 10, 3 );
/**
* Filter the cart template path to use cart.php in this plugin instead of the one in WooCommerce.
*
* @param string $template Default template file path.
* @param string $template_name Template file slug.
* @param string $template_path Template file name.
*
* @return string The new Template file path.
*/
function intercept_wc_template( $template, $template_name, $template_path ) {
if ( 'cart.php' === basename( $template ) ) {
$template = trailingslashit( plugin_dir_path( __FILE__ ) ) . 'woocommerce/cart/cart.php';
} elseif ( 'form-billing.php' === basename( $template ) ) {
$template = trailingslashit( plugin_dir_path( __FILE__ ) ) . 'woocommerce/checkout/form-billing.php';
}
return $template;
}
Here we are overriding cart.php
and form-billing.php
.
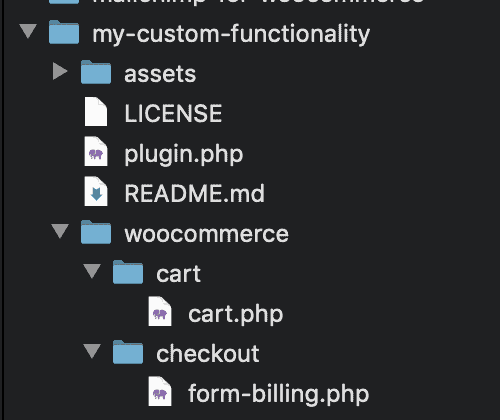
What if you want to override a number of files and do not want to specify the file names/paths each time in the code?
Use the following dynamic code instead:
add_filter( 'woocommerce_locate_template', 'intercept_wc_template', 10, 3 );
/**
* Filter the cart template path to use cart.php in this plugin instead of the one in WooCommerce.
*
* @param string $template Default template file path.
* @param string $template_name Template file slug.
* @param string $template_path Template file name.
*
* @return string The new Template file path.
*/
function intercept_wc_template( $template, $template_name, $template_path ) {
$template_directory = trailingslashit( plugin_dir_path( __FILE__ ) ) . 'woocommerce/';
$path = $template_directory . $template_name;
return file_exists( $path ) ? $path : $template;
}
After this, you can override any WooCommerce template simply by copying it to corresponding directory in your custom plugin’s woocommerce directory and editing it.
Credits:
https://stackoverflow.com/a/43776583/778809
https://pluginrepublic.com/override-woocommerce-template-plugin/